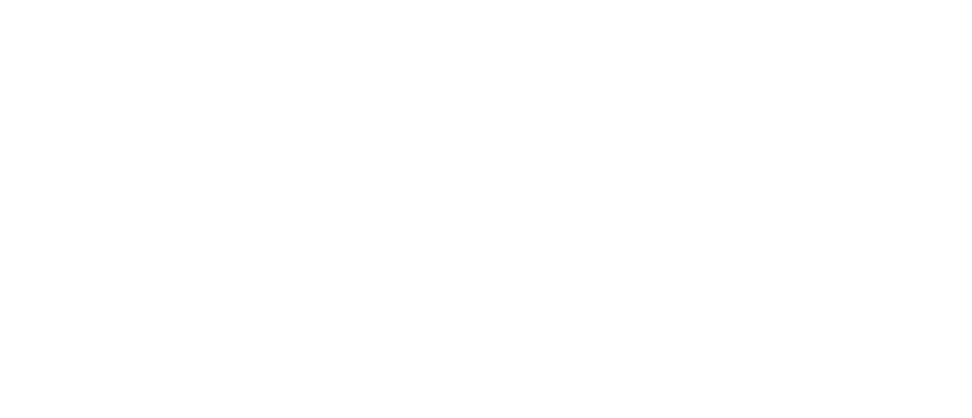
Develop, Extend, and Connect Aprimo with Ease
Explore powerful APIs, Webhooks, and SDKs to integrate your marketing technology stack.
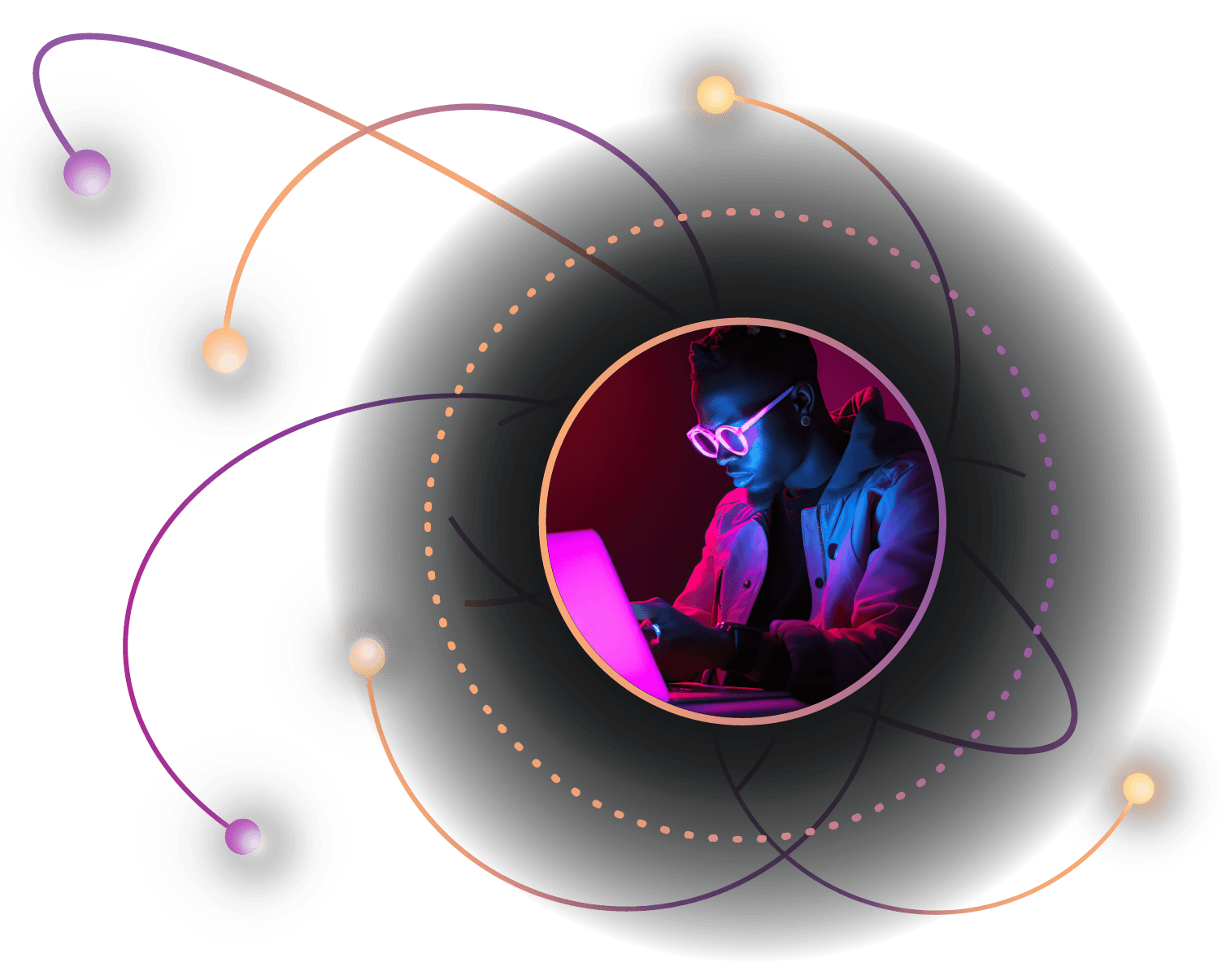
Discover Powerful APIs & Developer Tools
REST APIs
Access Aprimo’s core functionality programmatically through RESTful endpoints for seamless integration.
Learn MoreWebhooks
Receive near real-time HTTP callouts with event-driven triggers, such as updates to content.
Learn MoreContent Selector SDK
Leverage Aprimo’s Content Selector directly in another application for picking assets or public links with user-level authorization provided.
Learn MoreUI Extensibility
Place pagehooks to extend Aprimo's UI, enabling user-driven callouts with optional redirection to external pages.
Learn MoreAnalytics APIs
Retrieve and analyze data to gain actionable insights by accessing reports via API, exporting data automatically, or leveraging an aggregate analytics API for measuring asset engagement.
Learn MoreTutorials
Onboard as a developer with tutorials covering the most common integration use cases requested.
Learn More